Working with APIs in Google Colab is a common practice for data scientists, researchers, and developers. However, handling API keys, which are essentially passwords granting access to these services, requires careful consideration. Directly embedding API keys in your code or storing them as plain environment variables within your Colab notebooks poses significant security risks. Google Colab’s “Secrets” feature offers a robust solution to this problem, providing a secure and convenient way to manage sensitive credentials. This comprehensive guide delves into the importance of protecting API keys, the vulnerabilities of traditional methods, and a detailed walkthrough of using Colab Secrets effectively.
Learning Objectives
- Learners will be able to securely store API keys and other sensitive data using Google Colab’s Secrets feature.
- Learners will be able to retrieve and utilize stored secrets within their Colab notebooks without exposing the actual values in their code.
- Learners will be able to integrate secrets as environment variables for use with libraries that require this method of authentication.
- Learners will be able to apply best practices for managing secrets, including naming conventions, access control, and secure updating.
This article was published as a part of the Data Science Blogathon.
Critical Need for API Key Protection
API keys are like digital keys to various services, allowing your applications to interact with them. If these keys fall into the wrong hands, the consequences can be severe:
- Unauthorized Access and Usage: Malicious actors could use your API keys to access services without your consent, potentially incurring unexpected costs or exceeding usage quotas.
- Data Breaches and Security Compromises: In some cases, compromised API keys could grant access to sensitive data or allow unauthorized modifications to your accounts.
- Reputational Damage: Security breaches can damage your reputation and erode trust among users and stakeholders.
Therefore, implementing robust security measures to protect API keys is paramount.
Why Use Secrets?
Storing API keys directly in your Colab notebooks or as standard environment variables exposes them to several vulnerabilities:
- Exposure in Shared Notebooks: If you share your notebook with collaborators or publish it publicly, your API keys become readily accessible to anyone who views the notebook.
- Version Control Risks: Committing notebooks containing API keys to version control systems like Git can inadvertently expose them to the public, as these repositories are often publicly accessible. Even private repositories can be vulnerable if access control is not properly configured.
- Difficult Key Rotation: Changing API keys becomes a cumbersome process if they are embedded throughout your code. You would need to manually update every instance of the key, increasing the risk of errors and inconsistencies.
Introducing Google Colab Secrets: A Secure Solution
Google Colab’s Secrets feature addresses these vulnerabilities by providing a secure and centralized way to manage sensitive information. Here’s how it enhances security:
- Encrypted Storage: Secrets are encrypted and stored securely on Google’s servers, protecting them from unauthorized access.
- Granular Access Control: You can control which notebooks have access to specific secrets, ensuring that only authorized notebooks can retrieve and use them.
- No Direct Exposure in Code: API keys are never directly embedded in your notebook code, eliminating the risk of accidental exposure through sharing or version control.
- Simplified Key Rotation: Updating an API key is as simple as modifying the secret value in the Secrets panel. All notebooks using that secret will automatically use the updated value.
Step-by-Step Guide to Using Colab Secrets
Here’s how to use secrets in Google Colab:
Step1: Access the Secrets Feature
- Open your Google Colab notebook.
- In the left-hand sidebar, you’ll find an icon that looks like a key. Click on it to open the “Secrets” panel.
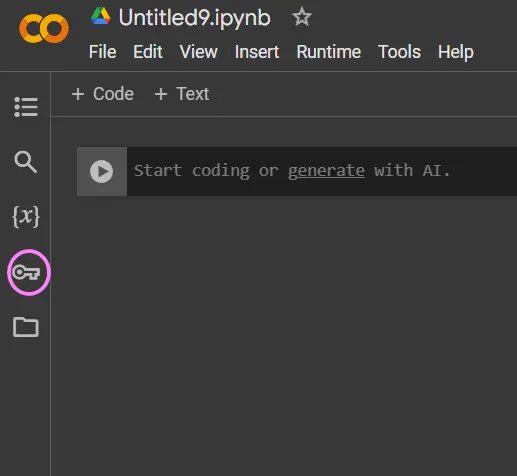
Step2: Create a New Secret
- Click on “Add a new secret”.
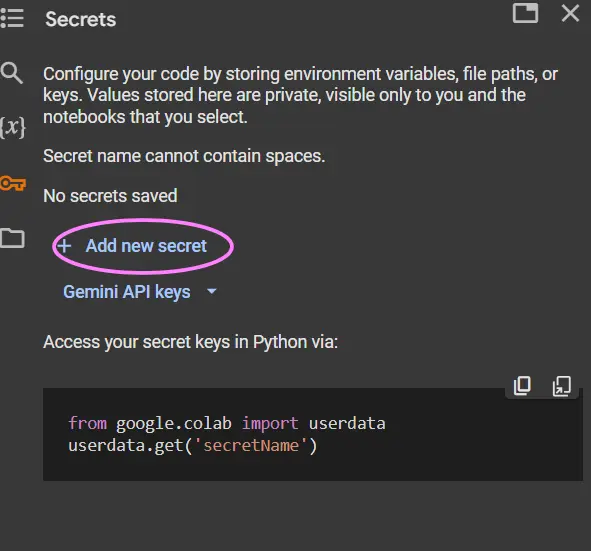
- Give your secret a descriptive name (e.g., “OPENAI_API_KEY”). Note that the name is permanent and cannot be changed later.
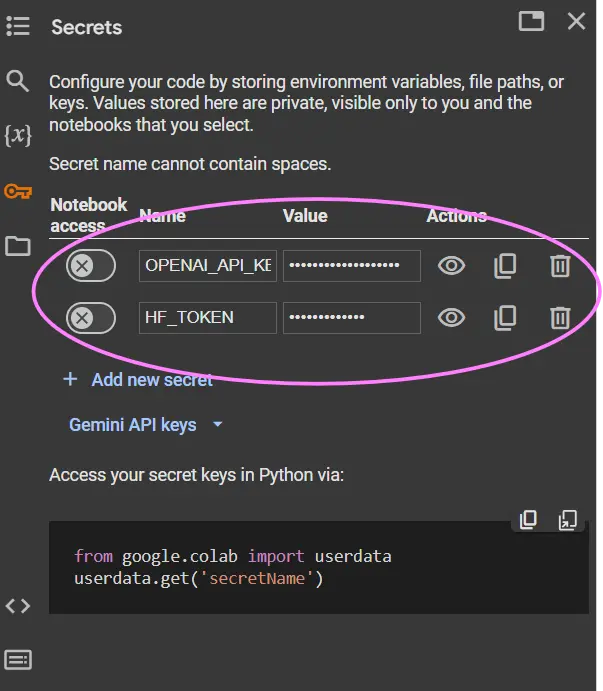
- Enter the actual API key value in the “Value” field.
- Click “Save”.
Step3: Grant Notebook Access
- Once the secret is created, you’ll see a toggle switch next to it.
- Make sure the toggle is enabled to grant the current notebook access to the secret.
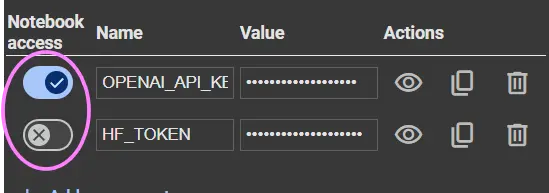
Step4: Use the Secret in Your Notebook
- To retrieve the secret value in your code, use the following code snippet:
from google.colab import userdata
api_key = userdata.get('OPENAI_API_KEY')
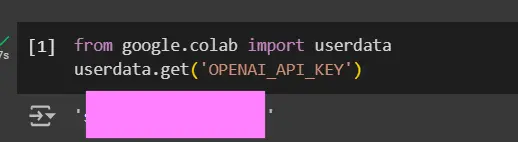
- Replace ‘OPENAI_API_KEY’ with the actual name of your secret.
- The userdata.get() function retrieves the secret value as a string. If your secret is a number, you’ll need to convert it accordingly (e.g., int(userdata.get(‘MY_NUMBER’))).
Step5: Using Secrets as Environment Variables
- Many Python libraries expect API keys to be set as environment variables. You can easily achieve this using the os module:

import os
from google.colab import userdata
os.environ["OPENAI_API_KEY"] = userdata.get('OPENAI_API_KEY')
# Now you can use the API key with libraries that rely on environment variables # Example: # import openai
# openai.api_key = os.getenv("OPENAI_API_KEY")
Best Practices for Managing Secrets
Below we will look into the best practices for managing secrets:
- Meaningful Secret Names: Use descriptive and consistent naming conventions for your secrets to easily identify and manage them.
- Regular Access Review: Periodically review which notebooks have access to your secrets and revoke access for notebooks that no longer require them.
- Careful Secret Updates: When updating an API key, update the corresponding secret value in the Secrets panel. Avoid deleting and recreating secrets unless absolutely necessary.
- Avoid Printing Secrets: Never print or display the actual secret value in your notebook output. This is a crucial security precaution.
- Principle of Least Privilege: Grant access to secrets only to the notebooks that absolutely need them. Avoid granting broad access unless necessary.
Conclusion
Using Google Colab’s Secrets feature is essential for maintaining the security of your API keys and other sensitive information. By following the guidelines outlined in this article, you can significantly reduce the risk of unauthorized access and ensure the integrity of your projects. Implementing these best practices will contribute to a more secure and efficient workflow when working with APIs in Google Colab.
Key Takeaways
- Directly embedding API keys in Google Colab notebooks is a significant security risk. Sharing notebooks or committing them to version control can expose these sensitive credentials.
- Google Colab’s Secrets feature provides a secure alternative for storing and managing API keys. Secrets are encrypted and accessed programmatically, preventing direct exposure in code.
- Secrets can be easily retrieved within Colab notebooks using the userdata.get() function and integrated as environment variables. This allows seamless use with various libraries and APIs.
- Following best practices for secret management, such as using descriptive names and regularly reviewing access, is crucial for maintaining security. This ensures only authorized notebooks can access necessary credentials.
Frequently Asked Questions
A. No. Secrets are stored securely by Google and are not included when you share your notebook. Others will need to create their own secrets with the same names if they want to run the code.
A. No, the name of a secret cannot be changed after creation. If you need a different name, you’ll have to create a new secret and delete the old one.
A. Simply go to the Secrets panel, find the secret you want to update, and change the value in the “Value” field. The change will be reflected in any notebooks that use that secret.
A. While there’s no explicitly documented limit, creating an excessive number of secrets might impact performance. It’s best to manage your secrets efficiently and avoid creating unnecessary ones.
A. No, deleting a notebook does not delete the associated secrets. You must manually delete secrets from the Secrets panel if you no longer need them. This is an important security feature to prevent accidental data loss.
The media shown in this article is not owned by Analytics Vidhya and is used at the Author’s discretion.